It is a human tendency to deviate from the Goal, and this happens mostly because they don't have a clear understanding of the path they are in. Believe me, setting up a goal have no value if you don't know how to reach there. So, In this article, I am going to explain the path that we will take to learn ASP.NET Core 5+.
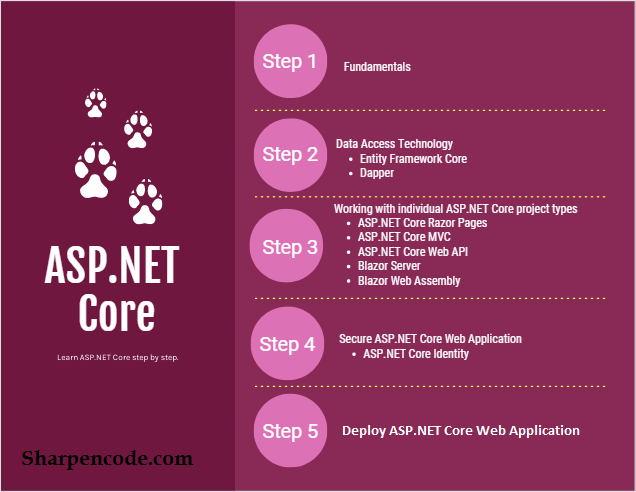
Step 1. Fundamentals
The fundamental section will cover all the key concepts, that we need to learn to create a web application using ASP.NET Core 5. The fundamental topics will be applicable to each ASP.NET Core project type. I have already discussed all the 5 project types that ASP.NET Core offers in the previous article.
First, we will create an empty ASP.NET Core application and will try to understand the directory structure. we will discuss the purpose of each file and folder that gets generated when we create an ASP.NET Core application using .NET Core CLI or Visual Studio.
Please note that the fundamental topics will be applicable to each ASP.NET Core project type, so I highly recommend you to learn them well. From an interview perspective, this section will be the most important for you.
Step 2. Data Access
A web application without data is nearly impossible to exist. You create a web application to display some information, it doesn't matter you are creating an E-Commerce website or a Blog, you will always need some data, most often we store those data in a database. But, a database can only understand the SQL, and you can't fire an SQL query directly from your application. So, you need a mediator that sits in-between your application and the Database.
We can use classic ADO.NET as the data access technology to interact with the database, but we always need to map those data to some classes and objects that are always time-consuming and hectic. For, this reason we use ORMs. I have already discussed the ORM here, please have a look at that.
As a part of Data Access technology we will use
• Entity Framework Core
• Dapper
EF core is a full ORM, while Dapper is a micro ORM, Micro ORMs are lightweight ORM, and are usually limited in features, but performing faster than full-featured ORM, this means Micro-ORMs suppose to do the same job of mapping table to object, but it sacrifices a number of features to have a better performance. For example, Entity Framework Core has the ability to generate databases and tables based on POCO classes or vice versa but Micro-ORMs can't do that but works great for Stored procedures or in-line SQL queries.
Don't worry we will learn everything about EF Core and Dapper in great detail with examples. We will write persistence Logic for our app using both EF Core and Dapper, and thus we will have two implementations for our Data Access Layer, not only that, but we will also learn to switch between those implementations using Dependency injection, as ASP.NET Core has built-in support for DI.
As a side note, Entity Framework is the official data access technology from Microsoft but Dapper is a third-party tool, Dapper is from Stack Overflow.
Step 3. Working with individual ASP.NET Core project types.
Step 4 will be the largest module, here we will go through each ASP.NET Core project types one by one. First, we will discuss the ASP.NET Core Razor pages in detail, we will create a fully functional application, and will discuss the advanced topics related to the ASP.NET Core Razor pages. After completing the Razor pages we will do the same for all other project types that are ASP.NET Core MVC, ASP.NET Core Web API, and ASP.NET Core Blazor.
Step 4. Learn to secure ASP.NET Core applications.
Security has always been a major concern for developers, but ASP.NET Core enables developers to easily configure and manage security for their apps. ASP.NET Core contains features for managing authentication, authorization, data protection, HTTPS enforcement, app secrets, XSRF/CSRF prevention, and CORS management. These security features allow us to build robust yet secure ASP.NET Core apps.
Here we will learn
• Cookie based Authentication
• Role-based and Claims based Authorization
• Authentication with OAuth e.g Facebook and Google
• Token based Authentication for REST APIs.
• Prevent Cross-Site Request Forgery (XSRF/CSRF) attacks
• Prevent open redirect attacks
• Prevent Cross-Site Scripting (XSS)
• Enable Cross-Origin Requests (CORS)
Step 5. Understanding the ASP.NET Core Application Life Cycle and Hosting the application on IIS
Here, we will discuss everything that happens in between when a user issues a request from his browser and gets the response from the server. Having knowledge of the request-response cycle always helps in creating a robust and maintainable application and understanding the technology better. We will learn to host our application on IIS, but will also discuss hosting ASP.NET Core application over Linux environment with Apache as a reverse proxy server and Kestrel as the main request processing server.
Setting up the development environment
Before starting the journey to ASP.NET Core, it becomes necessary to set up the development environment. In order to develop and run an ASP.NET Core application, we need to download and install .NET SDK and .NET Runtime respectively. But, we don't need to install the .NET runtime explicitly after installing .NET SDK because the .NET SDK includes the .NET runtime.
Follow the below steps to set up the ASP.NET Core development environment.
1. Download and Install the .NET SDK
The .NET SDK (Software development KIT) includes the .NET runtime and command line tools required to build and run .NET 5 applications.
Download
Download the .NET SDK from https://dotnet.microsoft.com/download.
Install
Install the .NET SDK by simply opening the downloaded installer and then following the prompts.
Verify Installation
Verify that the .NET SDK was installed successfully by running the command dotnet or dotnet --version.on command prompt.
2. Download and Install Visual Studio Code (VS Code)
VS Code is a free code editor for building cloud and web applications and runs equally well on Windows, Mac and Linux.
Download
Download VS Code from https://code.visualstudio.com/.
Install
Install Visual Studio Code by simply opening the downloaded setup file and then following the prompts.
3. Install the C# Extension for VS Code
The C# extension adds support to VS Code for developing ASP.NET Core applications.
Install
Open Visual Studio Code and follow below steps to install the C# extension:
1. open Extensions view by pressing Ctrl+Shift+X
2. Type"c#" in the extensions search box.
3. Select "C# for Visual Studio Code (powered by OmniSharp)".
4. Install the C# extension by clicking the "Install" button.
If you are a visual studio lover
Visual Studio is an IDE (Integrated development environment) for developing all types of .NET application (Desktop/Mobile/Web).Download and Install Visual Studio 2019
Download
Download Visual Studio from https://visualstudio.microsoft.com
Install
Install Visual Studio by opening the downloaded setup file and following the prompts.
.NET SDK in Detail
The .NET SDK is a set of libraries and tools for developing and running .NET applications, when we download the SDK, we automatically get the Runtime (CLR) with it.
The .NET SDK includes the following components
1.The .NET CLI (command line tool) that we can use for local development.
2. The .NET drivers, that support executing CLI commands and running framework-dependent apps.
3.Roslyn (C# and VB compiler)
4.MSBuild, the Microsoft build engine for building applications.
5.The .NET runtime (CLR), that provides type system, assembly loading, Garbage collection, native interop and some other services. The .NET runtime includes both ASP.NET Core runtime and Desktop runtime.
• ASP.NET Core Runtime provides basic services for web apps, IoT apps, and mobile backends.
• Desktop Runtime provides basic services for Windows Forms and WPF.
6.The runtime libraries, also known as framework libraries or base class libraries. These libraries provides implementations for many general purpose and workloads (ASP.NET, WPF, WinForms) specific types and utility functionalities. Some examples of types and utilities defined in .NET runtime libraries are :-
Types
• Primitive types : System.Boolen and System.Int32
• Collections : Syetem.Collections.Generic.List
• DataTypes :System.Data.DataSet and System.Data.DataTable.
Utilities
• Network Utility : System.Net.Http.HttpClient
• File and stream IO Utility : System.IO.FileStream and System.IO.TextWriter.
• Serialization Utility : System.Text.Json.JsonSerializer and System.Xml.Serialization.XmlSerializer.